In the ever-evolving world of programming, having a comprehensive cheat sheet can significantly enhance your coding efficiency and effectiveness. This article provides an extensive Python 3 cheat sheet in PDF format, designed to be a go-to resource for both beginners and experienced developers. Whether you’re brushing up on your skills or diving into Python 3 for the first time, this guide covers all the essential aspects of the language.
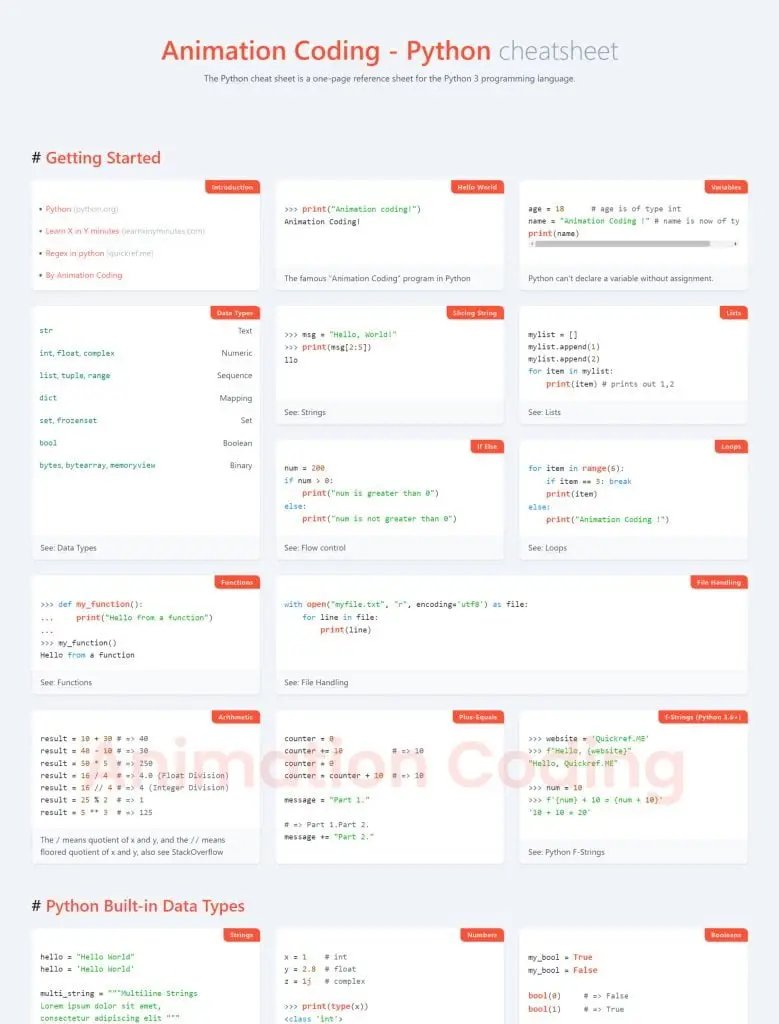
Table of Contents
- Introduction to Python 3
- Basic Syntax and Structure
- Data Types and Variables
- Operators and Expressions
- Control Flow Statements
- Functions and Modules
- File Handling
- Exception Handling
- Object-Oriented Programming
- Libraries and Frameworks
- Common Built-in Functions
- Conclusion
Introduction to Python 3
Python 3 is a powerful, versatile, and easy-to-learn programming language that is widely used for web development, data analysis, artificial intelligence, scientific computing, and more. Its simplicity and readability make it an ideal choice for both beginners and seasoned programmers. With a vast standard library and a supportive community, Python 3 continues to grow in popularity.
Basic Syntax and Structure
Comments
Comments in Python are indicated by the #
symbol. Multi-line comments can be achieved using triple quotes ('''
or """
).
# This is a single-line comment """ This is a multi-line comment """
Indentation
Python uses indentation to define blocks of code. Proper indentation is crucial as it affects the code’s execution.
if True: print("Hello, World!")
Variables and Assignments
Variables in Python do not require explicit declaration to reserve memory space. The assignment is done using the =
operator.
x = 5 y = "Hello"
Data Types and Variables
Numeric Types
- int: Integer values
- float: Floating-point values
- complex: Complex numbers
num1 = 10 # int num2 = 10.5 # float num3 = 1 + 2j # complex
Strings
Strings are arrays of bytes representing Unicode characters. They can be enclosed in single, double, or triple quotes.
str1 = 'Hello' str2 = "World" str3 = '''Python 3'''
Lists
Lists are ordered, mutable collections of items.
my_list = [1, 2, 3, 4, 5]
Tuples
Tuples are ordered, immutable collections of items.
my_tuple = (1, 2, 3, 4, 5)
Dictionaries
Dictionaries are unordered collections of key-value pairs.
my_dict = {'name': 'John', 'age': 25}
Sets
Sets are unordered collections of unique items.
my_set = {1, 2, 3, 4, 5}
Operators and Expressions
Arithmetic Operators
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus**
: Exponentiation//
: Floor division
a = 10 b = 3 print(a + b) # 13 print(a - b) # 7 print(a * b) # 30 print(a / b) # 3.3333333333333335 print(a % b) # 1 print(a ** b) # 1000 print(a // b) # 3
Comparison Operators
==
: Equal!=
: Not equal>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
x = 5 y = 3 print(x == y) # False print(x != y) # True print(x > y) # True print(x < y) # False print(x >= y) # True print(x <= y) # False
Logical Operators
and
: Logical ANDor
: Logical ORnot
: Logical NOT
a = True b = False print(a and b) # False print(a or b) # True print(not a) # False
Control Flow Statements
If Statements
x = 10 if x > 5: print("x is greater than 5") elif x == 5: print("x is equal to 5") else: print("x is less than 5")
For Loops
for i in range(5): print(i)
While Loops
count = 0 while count < 5: print(count) count += 1
Functions and Modules
Defining Functions
Functions are defined using the def
keyword.
def greet(name): return f"Hello, {name}!"
Importing Modules
Modules can be imported using the import
keyword.
import math print(math.sqrt(16))
Lambda Functions
Lambda functions are small anonymous functions defined using the lambda
keyword.
square = lambda x: x * x print(square(5)) # 25
File Handling
Python provides various functions for file operations.
Opening a File
file = open("example.txt", "r")
Reading a File
content = file.read() print(content) file.close()
Writing to a File
file = open("example.txt", "w") file.write("Hello, World!") file.close()
Exception Handling
Exception handling in Python is done using the try
, except
, else
, and finally
blocks.
try: x = 1 / 0 except ZeroDivisionError: print("Cannot divide by zero") else: print("No exceptions occurred") finally: print("This will execute no matter what")
Object-Oriented Programming
Python supports OOP, which includes the use of classes and objects.
Creating a Class
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): return "Woof!" my_dog = Dog("Buddy", 3) print(my_dog.bark()) # Woof!
Inheritance
class Animal: def __init__(self, name): self.name = name def speak(self): raise NotImplementedError class Dog(Animal): def speak(self): return "Woof!" my_dog = Dog("Buddy") print(my_dog.speak()) # Woof!
Libraries and Frameworks
Python’s extensive libraries and frameworks facilitate various functionalities.
NumPy
NumPy is a library for numerical computations.
import numpy as np arr = np.array([1, 2, 3, 4, 5]) print(arr)
Pandas
Pandas is used for data manipulation and analysis.
import pandas as pd data = {'Name': ['John', 'Anna', 'Peter', 'Linda'], 'Age': [28, 24, 35, 32]} df = pd.DataFrame(data) print(df)
Django
Django is a high-level web framework.
# Sample view in Django from django.http import HttpResponse def hello(request): return HttpResponse("Hello, World!")
Common Built-in Functions
print()
Prints to the console.
print("Hello, World!")
len()
Returns the length of an object.
print(len("Hello")) # 5
type()
Returns the type of an object.
print(type(5)) # <class 'int'>
range()
Generates a sequence of numbers.
for i in range(5): print(i)
This comprehensive Python 3 cheat sheet is designed to be an essential resource for anyone working with Python. From basic syntax and data types to advanced topics like object-oriented programming and popular libraries, this guide covers it all. We hope this cheat sheet will help you enhance your Python programming skills and become more efficient in your coding endeavors.
If you want to read more information about how to boost traffic on your Website just visit –> The Insider’s Views “https://www.theinsidersviews.com/search/label/SEO”.